I recently worked on building an automation testing framework for our testers to use. I decided to turn the framework into a generic solution that can be used to automate any website or API. In this post, I’m going to explain how the solution is structured, how it works and some of the concepts in the framework. The full solution can be found here.
Specflow will be used to allow writing your test cases in the easy to understand Gherkin Syntax (Given-When-Then). Specflow is a behaviour Driven Development (BDD) for .NET. More details on Specflow can be found here.
We will also use Selenium WebDriver to automate browsers and drive the UI. Selenium is a library that enable and support the automation of web browsers. More details on Selenium can be found here.
The solution consists of 4 projects.
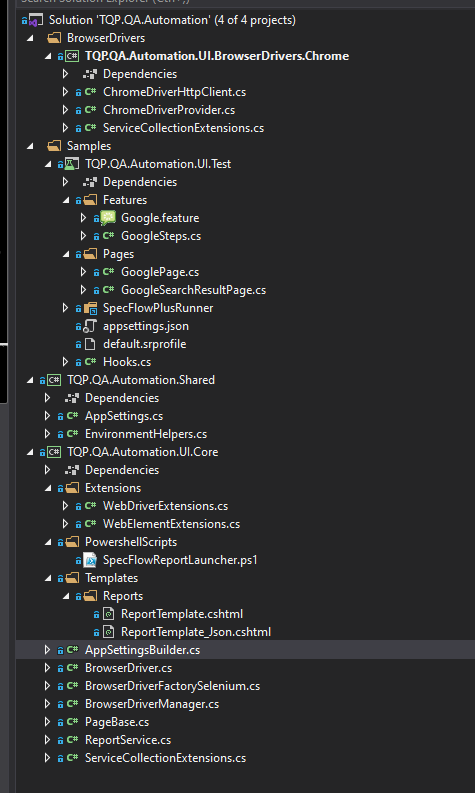
TQP.QA.Automation.Shared
This project contains everything that is shared across all other projects such as appsettings and helper/utility classes.
The AppSettings allows you to set the hosts for your tests, configure the browser and additional developer configurations. The browser configuration allows you to specify the browser type (only Chrome is supported at the moment, other types will be in the future) and how you want to run the browser. You can run the browser driver locally or as a remote driver. There’s also a setting to run the browser in headless.
TQP.QA.Automation.UI.BrowserDrivers.Chrome
This project contains Chrome browser driver. The ChromeDriverHttpClient allows getting the latest browser driver version given a Chrome version as well as downloading browser driver. This is very important. In the past, we have the browser drivers for different Chrome versions in the solution. This quickly becomes very annoying as Chrome automatically updates and we would have to manually download a new browser driver version for it. The ChromeDriverProvider will detect the currently installed version of Chrome and check if the browser driver is installed for that version. It will automatically download the new browser driver for Chrome if not found.
TQP.QA.Automation.UI.Core
This project is the core library for the solution. It has PageBase which is used to build page object models, more on this later. It also has extension methods on IWebDriver and IWebElement to help finding elements on a web page easier. It contains the template for tests report which you can customise and a ReportService which launches the report when tests finish running.
TQP.QA.Automation.UI.Test
This is a sample test project to show how the tests are written. This project tests searching JustSimplyCode on Google. It has a feature file with one test scenario as followed.
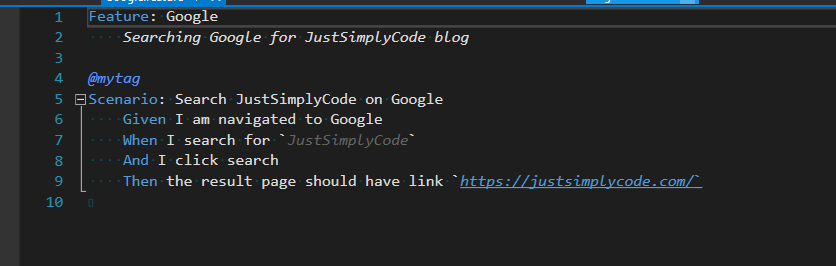
This will allow you to add specific SpecFlow files (like the feature file above) and also give you some IDE features.
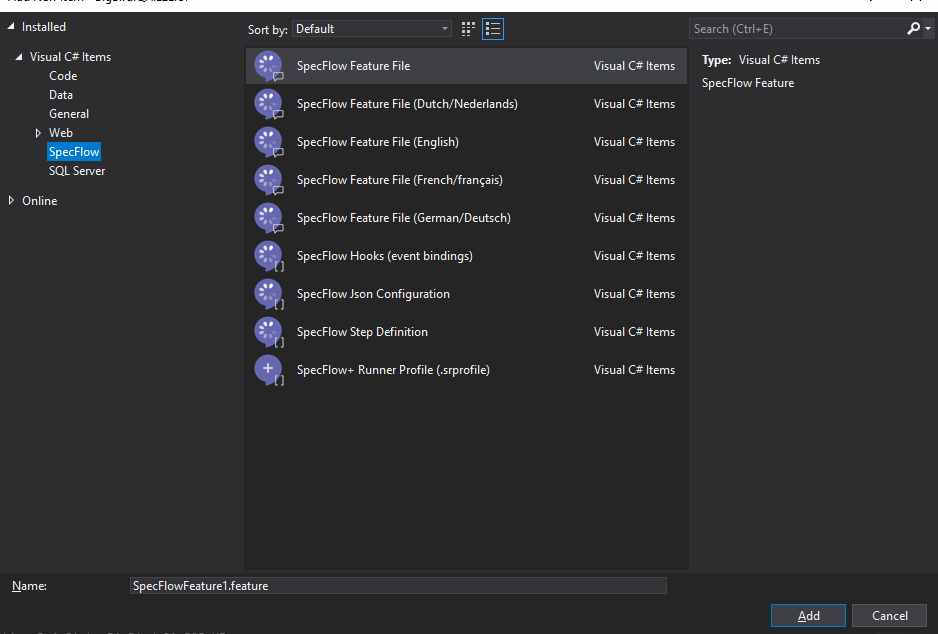
For test execution, we use the SpecFlow+ Runner. This is free (and free forever) however it does require activation. More information can be found here.
Writing tests
- Create a feature file (see the screenshot above about how to do this via the Visual Studio extension)
- Write a scenario in your feature file (BDD, Given-When-Then)
- Right-click in on the feature file editor (in blank space) and click “Generate Step Definitions”
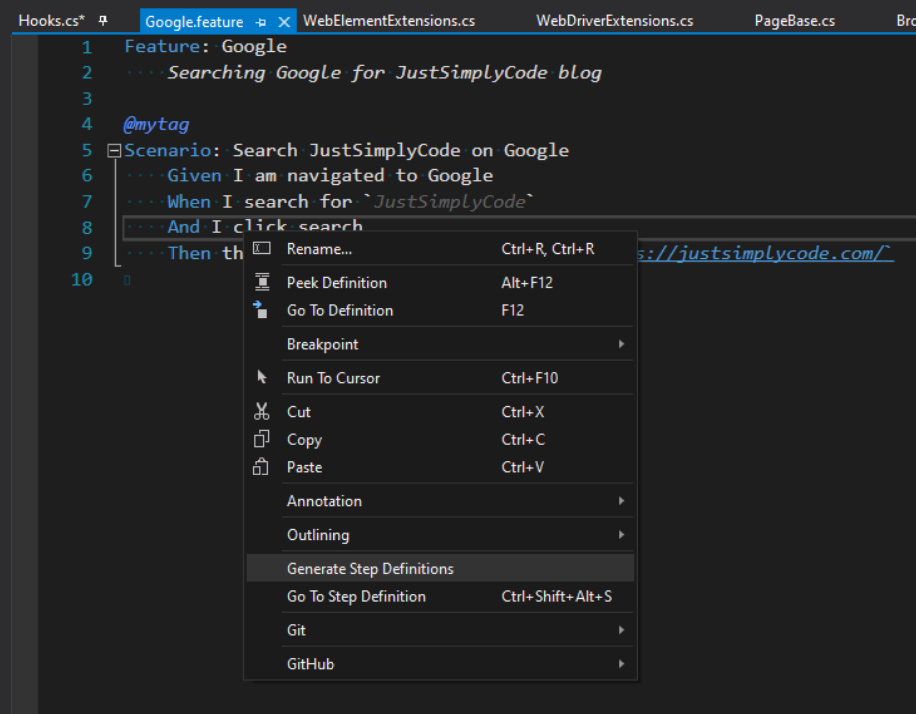
- This will give you a copy of the options, generally it is easiest to use the “Copy methods to clipboard” and paste them into a new manually created file class
- The standard convention is to create the new file in the same location as the feature file and name it similarly. E.G Google.feature & GoogleSteps.cs
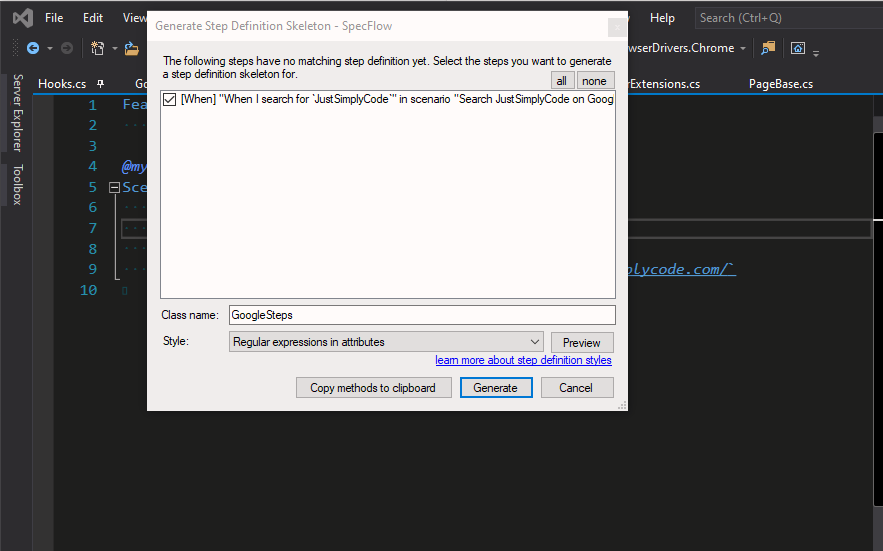
There are two additional things to do to your class file:
- Make sure it has a [Binding] element at the top of it
- If it has been generated using the obsolete static singleton ScenarioContext, refactor this and use DI
From this
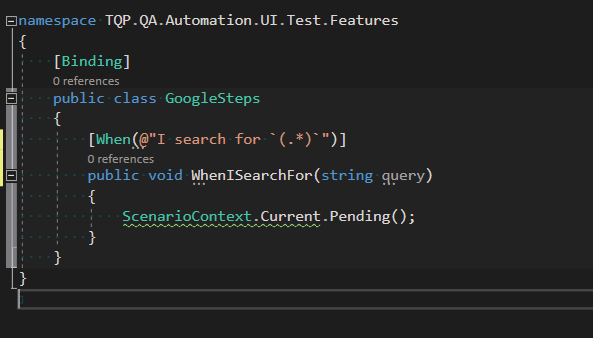
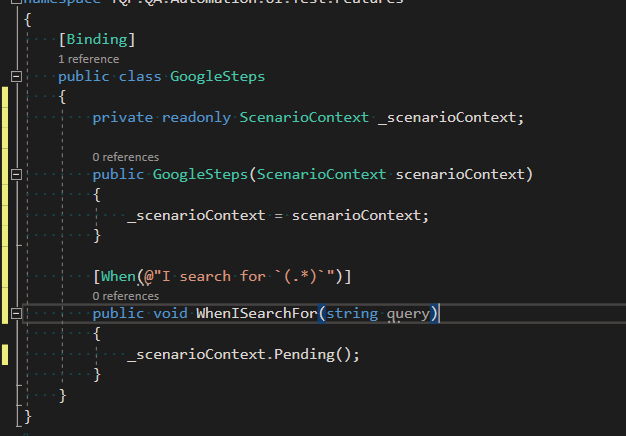
Pending is a convention that essentially means not implemented, this is where you need to add your own code to support the step.
Page Object Models
Page object models are classes which model all the UI elements on a particular page. They include things like textboxes, labels, checkboxes, links etc. Think of these page object models as domain models for a page, similar concept to DDD domain models. Page object models need to implement PageBase to have access to WebDriver which is what you need to select different elements on the UI.
This is an example of a page object model for Google page
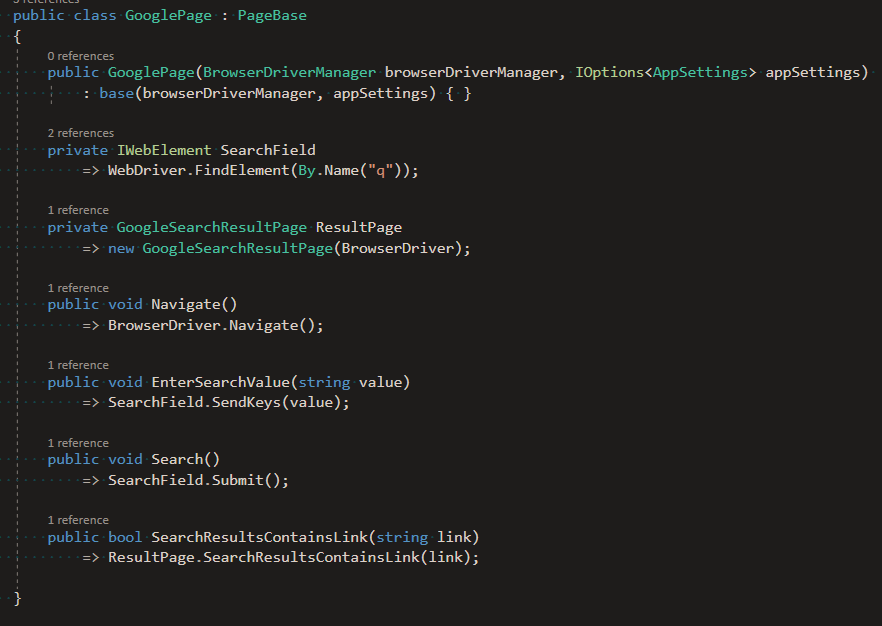
Conclusion
You can use the solution as the starting point for your Test Automation. It’s clean and easy to use. You can extend it to test API endpoints in BDD style too.