There are a couple ways to verify if 2 Json strings are equivalent.
Using DeepEquals on JObject
There’s a static function DeepEquals on JObject which compares the values of 2 tokens and all their descendant tokens. We can parse the Json strings into JObject and then use this function to compare them.
JObject object1 = JObject.Parse(@"
{
'Id': 1,
'ArticleName': 'Sample article name',
'PublishedDate': '10 Jan 2019',
'IsPublished': false
}");
JObject object2 = JObject.Parse(@"
{
'PublishedDate': '10 Jan 2019',
'ArticleName': 'Sample article name',
'IsPublished': false,
'Id': 1
}");
var result = JObject.DeepEquals(object1, object2);
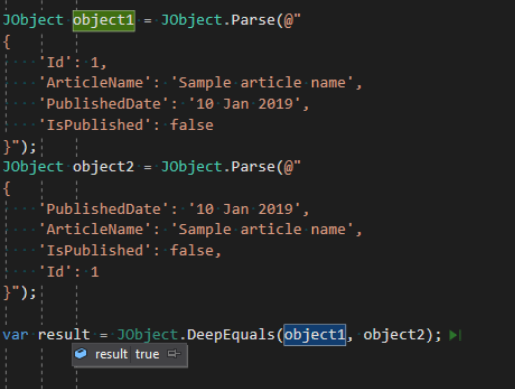
Using FluentAssertions
Another way is to the BeEquivalentTo() from FluentAssertions library to check if the 2 JObject variables are equivalent.
JObject object1 = JObject.Parse(@"
{
'Id': 1,
'ArticleName': 'Sample article name',
'PublishedDate': '10 Jan 2019',
'IsPublished': false
}");
JObject object2 = JObject.Parse(@"
{
'PublishedDate': '10 Jan 2019',
'ArticleName': 'Sample article name',
'IsPublished': false,
'Id': 1
}");
object1.Should().BeEquivalentTo(object2);