In my project at work, I come across a function where I have to deserialise a Json string to an anonymous object. For Json serialisation in this project, we always use the following Json serialisation settings.
var jsonSerializerSettings = new JsonSerializerSettings
{
ContractResolver = new CamelCasePropertyNamesContractResolver(),
NullValueHandling = NullValueHandling.Ignore,
Formatting = Formatting.None
};
I expect the result anonymous object would ignore null properties but they are included. See example below
var deserialisedObject = JsonConvert.DeserializeObject(@"{ 'PropertyA':'111', 'PropertyB': null}", jsonSerializerSettings);

This is also the same when I serialise it back to Json string.

I had a thought about it and it does make sense that the NullValueHandling.Ignore setting is ignored. Since it is deserialised to an anonymous object rather a strongly typed object, if the setting is not ignored then the result anonymous object is not the correct representation of the Json string and you would not be able to reference the null property.
However, what’s interesting is that if you serialise an anonymous object with null properties using the same settings (as in not serialising an deserialised object), the result Json string would ignore the null properties.
var sampleObject = new
{
PropertyA = "A",
PropertyB = 1,
PropertyC = (string)null
};
var jsonString = JsonConvert.SerializeObject(sampleObject, jsonSerializerSettings);
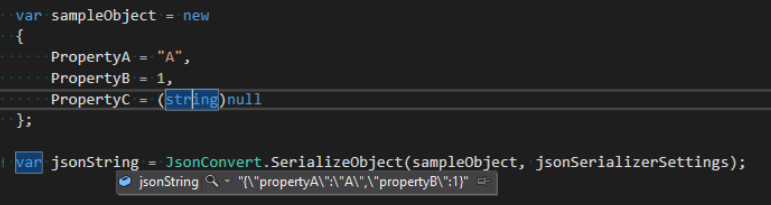
This now invalidates my thoughts on why the NullValueHandling.Ignore setting is ignored. I’m not sure why there’s an inconsistency in how the object is serialised using the same settings. I notice, however, that both JsonConvert.DeserializeObject() and JsonConvert.DeserializeObject<object>() return Newtonsoft.Json.Linq.JObject instead of object.
I’m guessing maybe there is something in the JObject that tells the Json serialiser to ignore the NullValueHandling setting. I could not find anything obvious when checking out all the properties on JObject though.