I have a need to override the default namespace and change the root name when serialising object to XML string. I could add XmlType attribute and change the namespace and add XmlRoot attribute for a different root name. However, we have different clients and each requires different namespace and root name. So I need to have a way to specify the default namespace and root name when serialising my object to XML string.
I have a method which takes a generic object with the namespace and root name to override. This method looks like the following:
public static string Serialise<T>(T payload, string rootName, string defaultNamespace)
{
var settings = new XmlWriterSettings
{
Indent = false,
NewLineHandling = NewLineHandling.None,
OmitXmlDeclaration = true
};
var namespaces = new XmlSerializerNamespaces();
namespaces.Add(string.Empty, defaultNamespace);
var stringwriter = new StringWriter();
var xmlWriter = XmlWriter.Create(stringwriter, settings);
var xmlSerialiser = new XmlSerializer(typeof(T), null, null, new XmlRootAttribute(rootName), defaultNamespace);
xmlSerialiser.Serialize(xmlWriter, payload, namespaces);
return stringwriter.ToString();
}
This is a sample class I want to deserialise
[XmlType("SomeClass", AnonymousType = true, Namespace = "http://abc.some.namespace")]
public class SomeClass
{
[XmlElement(Order = 0)]
public string Label { get; set; }
[XmlElement(Order = 1)]
public int Number { get; set; }
}
This is the result when I use that method

To deserialise the result string back to the object, I have the following method
public static T Deserialise<T>(string xmlString, string rootName, string defaultNamespace)
{
var xmlSerialiser = new XmlSerializer(typeof(T), null, null, new XmlRootAttribute(rootName), defaultNamespace);
return (T)xmlSerialiser.Deserialize(new StringReader(xmlString));
}
This is the result
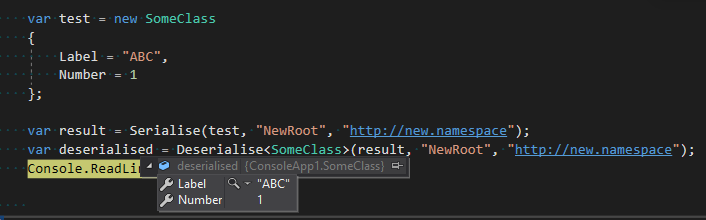